Use Python to scrape Reddit data by setting up your environment and using PRAW.
By the way, we're Bardeen, we build a free AI Agent for doing repetitive tasks.
If you're scraping Reddit, check out our AI Web Scraper. It automates data extraction without code, making your work easier.
Web scraping is a powerful technique for extracting data from websites, and Reddit, with its vast user-generated content, is a goldmine for valuable insights. In this step-by-step guide, we'll walk you through the process of web scraping Reddit using Python, covering essential tools, techniques, and best practices. Before diving in, it's crucial to understand the legal and ethical considerations surrounding web scraping and ensure compliance with Reddit's terms of service.
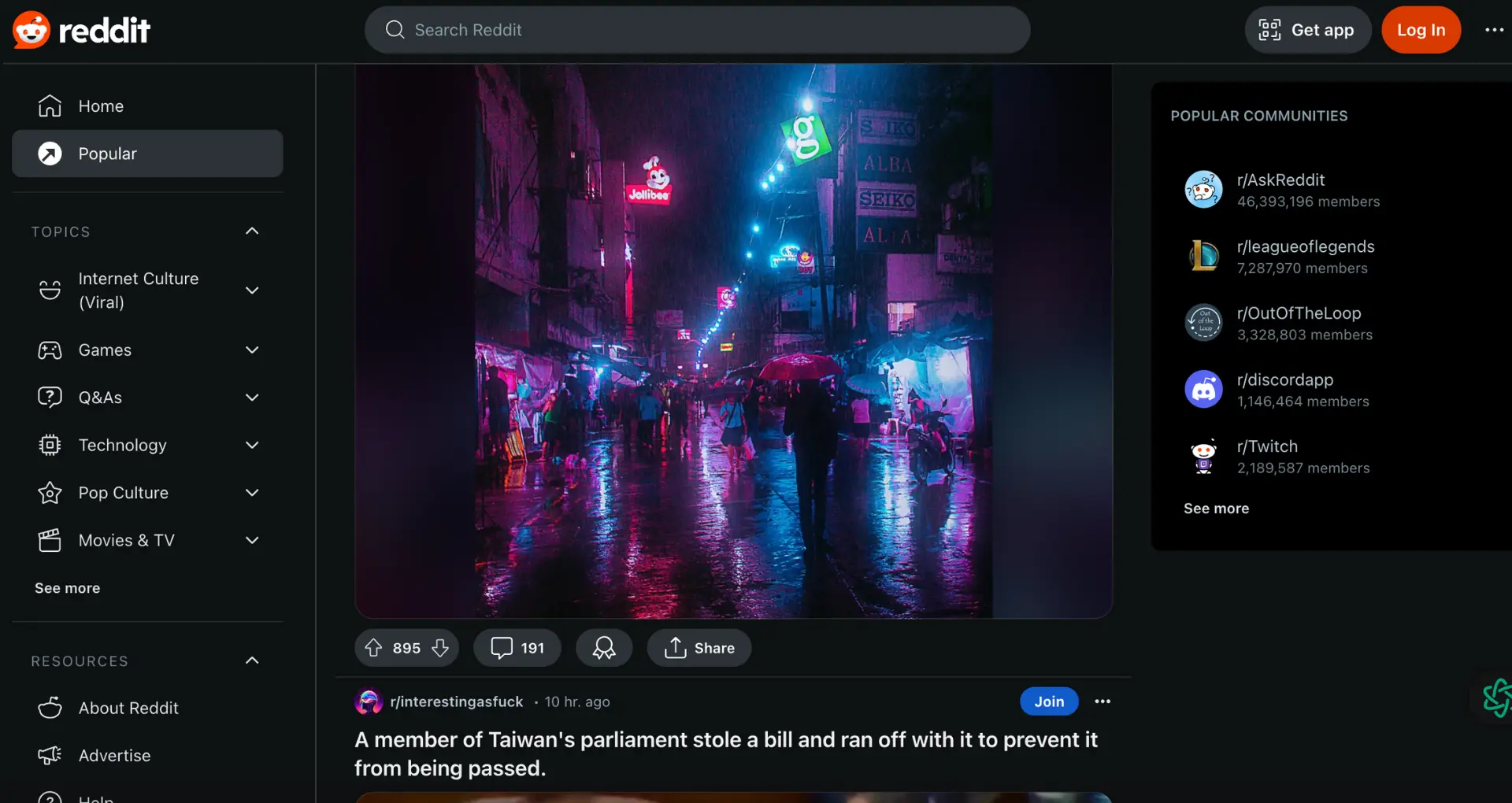
Introduction to Reddit Data Scraping
Web scraping is the process of extracting data from websites using automated tools or scripts. Reddit, being one of the largest online communities with a vast amount of user-generated content, is an invaluable source for data scraping. By scraping Reddit, you can gather insights, monitor trends, and analyze public sentiment on various topics.
However, before embarking on your Reddit scraping journey, it's crucial to understand the legal and ethical considerations involved. Always ensure that you comply with Reddit's terms of service and scrape responsibly. This means:
- Only scraping publicly available data
- Not overloading Reddit's servers with excessive requests
- Respecting user privacy and not scraping personal information
- Using the scraped data for legitimate purposes and not engaging in any malicious activities
By adhering to these guidelines, you can scrape Reddit data ethically and avoid any potential legal issues. Remember, responsible scraping is key to maintaining a healthy and trustworthy data ecosystem.

Setting Up Your Python Environment for Scraping
Before you start scraping Reddit data, you need to set up your Python environment. Here's a step-by-step guide:

- Install Python: Download and install the latest version of Python from the official website (python.org). Make sure to add Python to your system's PATH during the installation process.
- Create a Virtual Environment: It's recommended to create a virtual environment to manage your project's dependencies. Open a terminal or command prompt and navigate to your project directory. Run the following command to create a virtual environment named "venv":
python -m venv venv
- Activate the Virtual Environment: Activate the virtual environment by running the appropriate command based on your operating system:
- For Windows:
venv\Scripts\activate
- For macOS and Linux:
source venv/bin/activate
- For Windows:
- Install Required Libraries: With the virtual environment activated, install the necessary libraries for web scraping. Run the following commands to install PRAW, BeautifulSoup, and requests:
pip install praw beautifulsoup4 requests
PRAW (Python Reddit API Wrapper) is a Python package that simplifies accessing the Reddit API. It provides a convenient way to interact with Reddit's data programmatically.
BeautifulSoup is a popular library for parsing HTML and XML documents. It allows you to extract data from web pages by navigating the document tree and locating specific elements based on their tags, attributes, or text content.
The requests library is used for making HTTP requests to web servers. It simplifies the process of sending GET or POST requests and handling the response data.
With your Python environment set up and the required libraries installed, you're now ready to start scraping Reddit using Python!
Save time and focus on important tasks while Bardeen’s AI automates your scraping sequences. Check out our Reddit scraping playbook to simplify your workflow.
Utilizing PRAW to Extract Data from Reddit
PRAW (Python Reddit API Wrapper) is a powerful library that simplifies the process of accessing data from Reddit using Python. To start using PRAW, you need to register a Reddit application and obtain the necessary API credentials. Here's how:
- Go to the Reddit App Preferences page (https://www.reddit.com/prefs/apps) while logged in to your Reddit account.
- Scroll down to the "Developed Applications" section and click on the "Create App" or "Create Another App" button.
- Fill in the required information:
- Name: Choose a name for your application.
- App type: Select "Script."
- Description: Provide a brief description of your application.
- Redirect URI: Enter "http://localhost:8080" or any valid URL. This is not crucial for script applications.
- Click on the "Create App" button.
- After creating the app, you will see the "client_id" (under "personal use script") and "client_secret." Keep these credentials secure and do not share them publicly.
With the API credentials ready, you can now use PRAW to authenticate and access data from Reddit. Here's a basic example of how to authenticate and retrieve posts from a subreddit:
import praw
reddit = praw.Reddit(client_id="your_client_id",
client_secret="your_client_secret",
user_agent="your_user_agent")
subreddit = reddit.subreddit("subreddit_name")
for post in subreddit.hot(limit=10):
print(post.title)
In this example, we create a Reddit instance by providing the client_id, client_secret, and a user_agent string. Then, we specify the subreddit we want to access using reddit.subreddit()
. Finally, we iterate over the hot posts in the subreddit using subreddit.hot()
and print the title of each post.
PRAW provides various methods to access different types of data from Reddit, such as:
subreddit.hot()
: Retrieves the hot posts in a subreddit.subreddit.new()
: Retrieves the newest posts in a subreddit.subreddit.top()
: Retrieves the top posts in a subreddit.post.comments
: Accesses the comments of a specific post.reddit.redditor()
: Retrieves information about a specific user.
By leveraging PRAW's features, you can easily extract and analyze data from Reddit, including posts, comments, and user information. PRAW handles the authentication and API requests, allowing you to focus on processing and analyzing the data for your specific needs.
Advanced Scraping Techniques with BeautifulSoup and Selenium
When scraping dynamic web pages, you may encounter content that is generated or loaded dynamically using JavaScript. In such cases, using BeautifulSoup alone may not be sufficient. This is where Selenium comes into play.
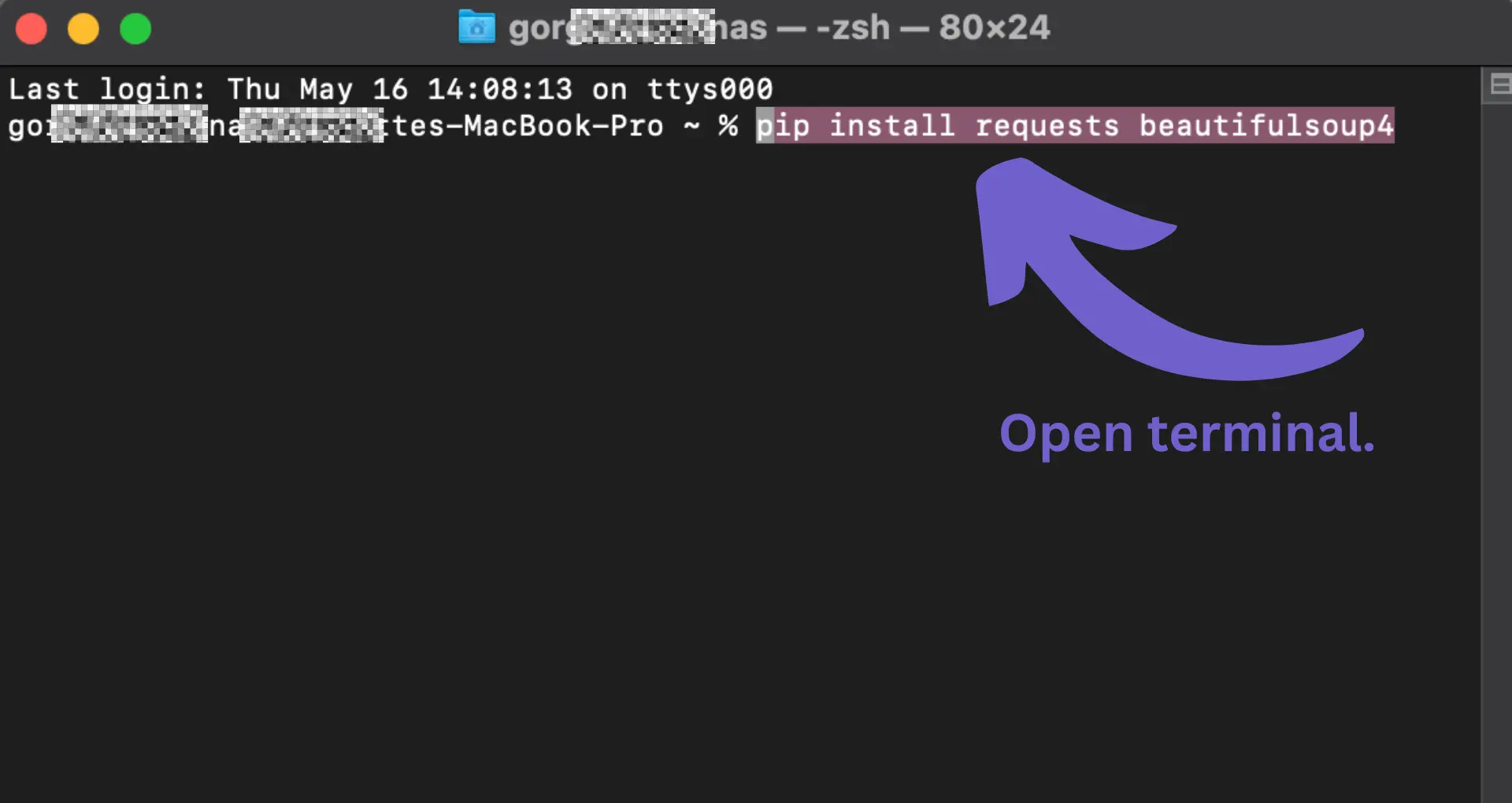
BeautifulSoup is a Python library that excels at parsing HTML and XML documents. It is ideal for scraping static web pages where the content is readily available in the HTML source. However, when dealing with dynamic content that requires interaction or is loaded asynchronously, BeautifulSoup falls short.
Selenium, on the other hand, is a powerful tool for automating web browsers. It allows you to simulate user interactions, such as clicking buttons, filling forms, and scrolling, making it suitable for scraping dynamic web pages. Selenium can wait for elements to load and can execute JavaScript, enabling you to access and extract content that is dynamically generated.
Here's an example of how you can use Selenium to scrape a dynamic web page:
from selenium import webdriver from selenium.webdriver.common.by import By driver = webdriver.Chrome() driver.get("https://example.com") # Wait for the dynamic content to load driver.implicitly_wait(10) # Find and extract the desired elements elements = driver.find_elements(By.CSS_SELECTOR, ".dynamic-content") for element in elements: print(element.text) driver.quit()
In this example, Selenium is used to launch a Chrome browser, navigate to the target URL, wait for the dynamic content to load, and then find and extract the desired elements using CSS selectors.
When scraping complex page structures on Reddit, you can leverage Selenium's capabilities to navigate through the page, interact with elements, and extract data. For example:
from selenium import webdriver from selenium.webdriver.common.by import By driver = webdriver.Chrome() driver.get("https://www.reddit.com/r/example") # Scroll to load more content driver.execute_script("window.scrollTo(0, document.body.scrollHeight);") # Find and extract post titles post_titles = driver.find_elements(By.CSS_SELECTOR, ".post-title") for title in post_titles: print(title.text) driver.quit()
In this scenario, Selenium is used to scroll the page to load more content and then extract the post titles from Reddit using CSS selectors.
By combining the parsing capabilities of BeautifulSoup with the dynamic interaction and JavaScript execution provided by Selenium, you can effectively scrape dynamic web pages and extract data from complex page structures on Reddit.
Save time and focus on important tasks while Bardeen’s AI automates your scraping sequences. Check out our Reddit scraping playbook to simplify your workflow.
Best Practices and Handling Common Scraping Challenges
When scraping data from Reddit or any other website, it's essential to follow best practices and handle common challenges to ensure a smooth and efficient scraping process. Here are some tips to keep in mind:
- Managing Request Headers: Websites often use request headers to identify and track scraping activities. To mimic human behavior and avoid detection, set appropriate headers such as User-Agent, Referer, and Accept-Language in your scraping requests.
- Handling Rate Limits: Many websites, including Reddit, impose rate limits to prevent excessive scraping. Respect these limits by adding delays between your requests using libraries like time.sleep() in Python. Monitor the response status codes and adapt your scraping rate accordingly.
- Using Proxies: If you encounter IP bans or restrictions, consider using proxies to rotate your IP address. You can use free or paid proxy services, or set up your own proxy server. Be cautious when using public proxies as they may be unreliable or slow.
- Efficient Data Parsing: When dealing with large volumes of scraped data, optimize your parsing techniques. Use libraries like BeautifulSoup or lxml for faster parsing of HTML and XML data. Avoid unnecessary string manipulation and leverage built-in methods for data extraction.
- Storing Scraped Data: Choose an appropriate format to store your scraped data based on your requirements. Common options include CSV files, JSON, or databases like SQLite or MongoDB. Ensure that your storage solution can handle the volume of data you anticipate and provides easy retrieval and analysis capabilities.
By implementing these best practices and being prepared to handle common scraping challenges, you can build robust and reliable scraping scripts for extracting data from Reddit or any other website.
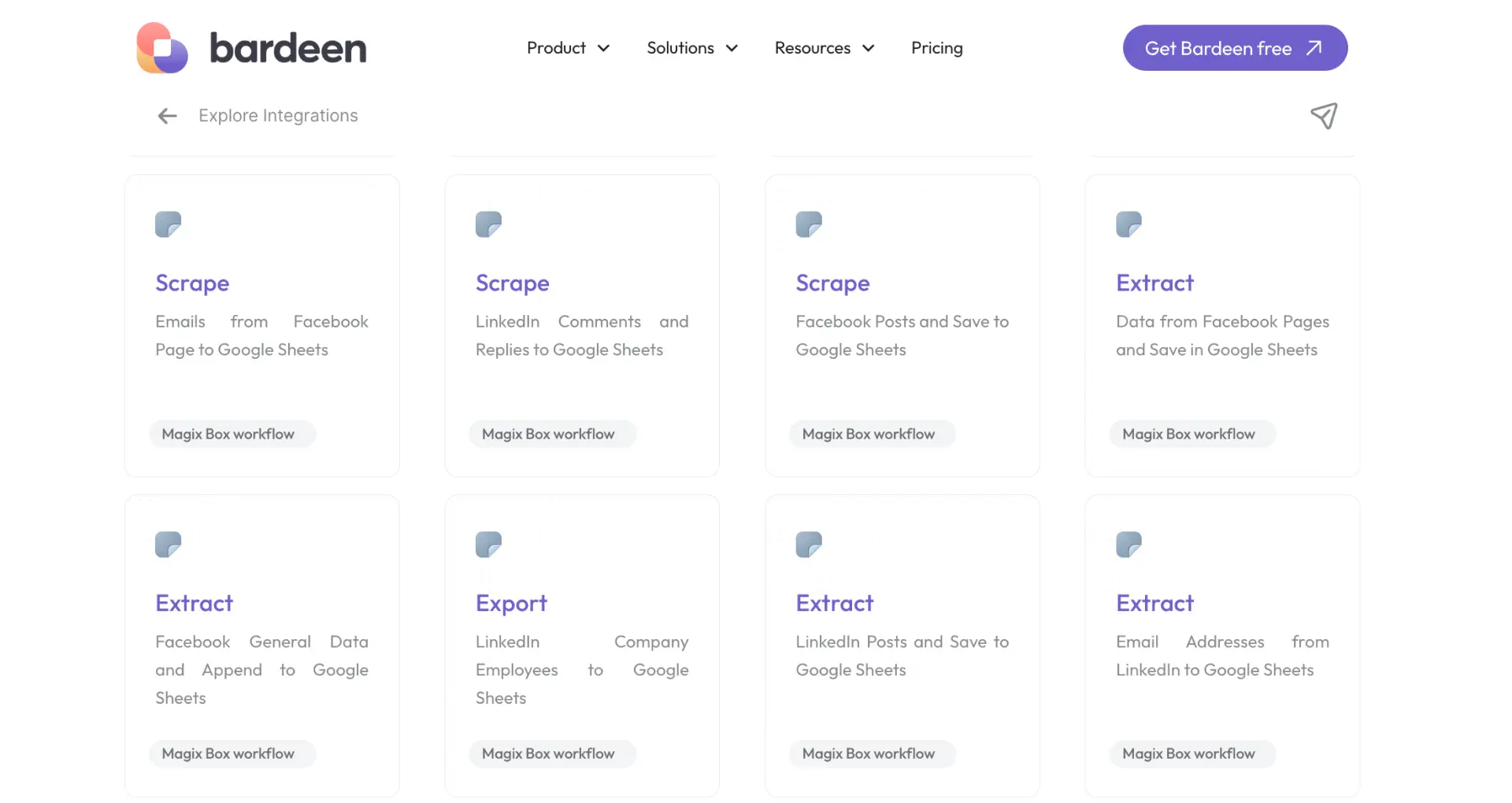
Automate Reddit Data Collection with Bardeen
Web scraping Reddit can either be done manually by coding with Python and its libraries or fully automated using Bardeen's Reddit integration. Automation is particularly beneficial for repetitive tasks such as gathering data for sentiment analysis, market research, or content aggregation without manual effort. Here are examples of automations that can be built with Bardeen using the provided playbooks:
- Get data from the currently opened Reddit post page: This playbook simplifies the process of collecting detailed information from a Reddit post, ideal for content curation and analysis.
- Get a list of post from the currently opened Reddit subreddit, home or search pages: Automate the extraction of posts from Reddit's subreddit, home, or search pages to streamline content discovery and market research.
- Get a summary of a Reddit post using openAI and save to Coda: This playbook offers a powerful way to summarize Reddit posts using OpenAI and save them to Coda for organized content planning or research.
Automating these tasks can save significant time and provide valuable insights efficiently. Get started by downloading the Bardeen app at Bardeen.ai/download