LinkedIn Data Scraping with Python: A Step-by-Step Guide
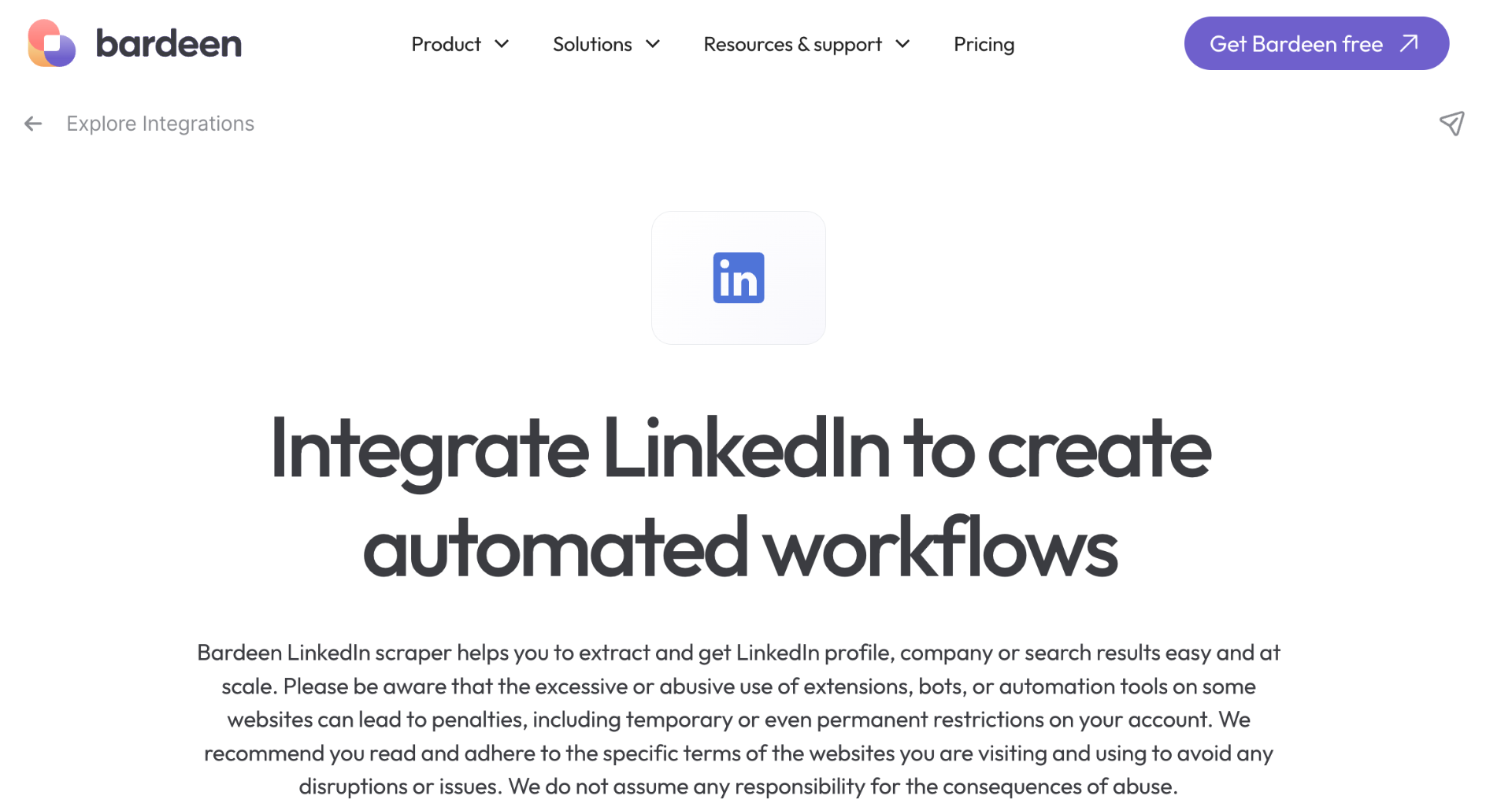
TL;DR
Scraping LinkedIn data using Python requires installing necessary libraries, understanding LinkedIn's site structure, and using tools like Selenium and Beautiful Soup for dynamic content and data extraction. This process enables the collection of profiles, jobs, and other LinkedIn data efficiently.
Mastering these techniques offers a comprehensive approach to LinkedIn data scraping.
For a simpler and code-free solution, automate your LinkedIn data extraction tasks with Bardeen.
In this step-by-step guide, we'll walk you through the process of scraping data from LinkedIn using Python, Selenium, and Beautiful Soup. We'll cover setting up your Python environment, understanding LinkedIn's dynamic site structure, automating browser interactions, extracting data, managing pagination, and saving scraped data. By the end of this guide, you'll have the tools and knowledge to responsibly and effectively gather data from LinkedIn.
Introduction
LinkedIn, the world's largest professional networking platform, is a goldmine of valuable data for businesses, researchers, and developers. From job listings and company profiles to user information and industry insights, LinkedIn holds a wealth of information that can be leveraged for various purposes. However, manually extracting this data can be a time-consuming and tedious task.
.png)
That's where web scraping comes in. By using Python, along with powerful libraries like Selenium and Beautiful Soup, you can automate the process of extracting data from LinkedIn. In this step-by-step guide, we'll walk you through the process of setting up your Python environment, understanding LinkedIn's site structure, automating browser interactions, extracting data, handling pagination, and saving the scraped data.
Whether you're a data analyst, researcher, or developer, this guide will provide you with the tools and knowledge to efficiently and responsibly scrape data from LinkedIn. By the end of this guide, you'll be able to create your own LinkedIn scraper and unlock the potential of this valuable data source. Let's get started!
Setting Up Your Python Environment for Scraping
Before diving into web scraping with Python, it's essential to set up your environment with the necessary tools and libraries. Here's a step-by-step guide to get you started:
- Install Python: Download and install the latest version of Python from the official website (python.org). Make sure to check the option to add Python to your system's PATH during the installation process.
- Set up a virtual environment (optional but recommended): Create a virtual environment to keep your project's dependencies isolated. Open your terminal and run the following commands:python -m venv myenv
source myenv/bin/activate - Install required libraries: With your virtual environment activated, install the essential libraries for web scraping:pip install requests beautifulsoup4 lxml selenium pandas
- Requests: A simple and elegant library for making HTTP requests.
- BeautifulSoup: A powerful library for parsing HTML and XML documents.
- lxml: A fast and feature-rich parser for processing XML and HTML.
- Selenium: A tool for automating web browsers, useful for scraping dynamic websites.
- Pandas: A data manipulation library that provides data structures for efficiently storing and analyzing scraped data.
.png)
By setting up a virtual environment, you can ensure that your project's dependencies are isolated from other Python projects on your system, avoiding potential conflicts.
With Python and the necessary libraries installed, you're now ready to start your web scraping journey using Python, Selenium, and Beautiful Soup.
Bardeen can help you save time on repetitive tasks. Try using our automate Google Search tasks playbook to see how simple automation can be.
Understanding LinkedIn's Dynamic Site Structure
To effectively scrape data from LinkedIn, it's crucial to understand the site's dynamic structure. LinkedIn heavily relies on JavaScript to render content, making it challenging for traditional web scraping techniques. Here's how you can navigate LinkedIn's structure:
- Open Chrome DevTools (right-click > Inspect) to examine LinkedIn's HTML structure.
- Identify key data points like job titles, company names, and other relevant information you want to extract.
- Notice that some content is loaded dynamically through AJAX calls and JavaScript rendering, which means the data might not be immediately available in the initial HTML source.
To locate the desired data, you can:
- Use the "Network" tab in Chrome DevTools to monitor AJAX requests and identify the endpoints that return the required data.
- Analyze the JavaScript code responsible for rendering the content to understand how the data is being populated.
By examining LinkedIn's dynamic structure, you can determine the optimal approach for extracting data using Selenium and Beautiful Soup. Selenium will help you interact with the dynamic elements, while Beautiful Soup will parse the rendered HTML to extract the desired information.
Automating Browser Interactions with Selenium
Selenium is a powerful tool for automating browser interactions, making it ideal for scraping dynamic websites like LinkedIn. Here's a step-by-step guide on setting up Selenium with WebDriver to interact with LinkedIn pages:
- Install Selenium: Use
pip install selenium
to install the Selenium library. - Download WebDriver: Selenium requires a WebDriver to interface with the chosen browser. For example, if using Chrome, download ChromeDriver.
- Set up Selenium: Import the necessary modules and initialize the WebDriver:
from selenium import webdriver driver=webdriver.Chrome('/path/to/chromedriver')
- Navigate to LinkedIn: Use the
driver.get()
method to navigate to the LinkedIn login page:driver.get('https://www.linkedin.com/login')
- Log in to LinkedIn: Find the email and password input fields using their HTML IDs, and use the
send_keys()
method to enter your credentials:email_field=driver.find_element_by_id('username') email_field.send_keys('your_email@example.com') password_field=driver.find_element_by_id('password') password_field.send_keys('your_password') password_field.submit()
- Navigate to relevant pages: Use Selenium's methods to click on links, scroll, and navigate to the desired pages containing the data you want to scrape.
Remember to handle any potential roadblocks, such as CAPTCHA or two-factor authentication, by incorporating appropriate waiting times or user input prompts.
With Selenium set up and logged in to LinkedIn, you can proceed to use Beautiful Soup to extract data from the dynamically loaded pages.
Bardeen can save you time automating repetitive tasks. Try our extract LinkedIn job data playbook to simplify your workflow.
Extracting Data with Beautiful Soup
Beautiful Soup is a powerful Python library for parsing HTML and XML documents. It allows you to extract specific data from web pages by navigating the document tree and searching for elements based on their tags, attributes, or text content. Here's how you can use Beautiful Soup to parse the HTML obtained from Selenium and extract structured data like job listings:
- Install Beautiful Soup:
pip install beautifulsoup4
- Import the library in your Python script:
from bs4 import BeautifulSoup
- Create a Beautiful Soup object by passing the HTML content and the parser type:
soup = BeautifulSoup(html_content, 'html.parser')
- Use Beautiful Soup's methods to locate specific elements:
find()
: Finds the first occurrence of a tag with specified attributes.find_all()
: Finds all occurrences of a tag with specified attributes.- CSS selectors: Use
select()
method with CSS selectors to locate elements.
- Extract data from the located elements:
get_text()
: Retrieves the text content of an element.get()
: Retrieves the value of a specified attribute.- Accessing tag attributes directly using square bracket notation.
.png)
Here's an example of extracting job listings from the HTML:
soup = BeautifulSoup(html_content, 'html.parser')
job_listings = soup.find_all('div', class_='job-listing')
for job in job_listings:
title = job.find('h2', class_='job-title').get_text()
company = job.find('span', class_='company').get_text()
location = job.find('span', class_='location').get_text()
print(f"Title: {title}")
print(f"Company: {company}")
print(f"Location: {location}")
print("---")
In this example, we locate all the div
elements with the class job-listing
using find_all()
. Then, for each job listing, we find the specific elements containing the job title, company, and location using find()
with the appropriate CSS class names. Finally, we extract the text content of those elements using get_text()
.
By leveraging Beautiful Soup's methods and CSS selectors, you can precisely pinpoint the data you need within the HTML structure and extract it efficiently.
Managing Data Pagination and Scraping Multiple Pages
Pagination is a common challenge when scraping data from websites, especially when dealing with large datasets that span across multiple pages. LinkedIn's job search results page often employs pagination or infinite scrolling to load additional job listings as the user scrolls down. To effectively scrape data from all available pages, you need to handle pagination programmatically. Here's how you can automate pagination handling and data collection across multiple pages:
- Identify the pagination mechanism:
- Check if the page uses numbered pagination links or a "Load More" button.
- Inspect the URL pattern for different pages to see if it follows a consistent format (e.g.,
https://www.linkedin.com/jobs/search/?start=25
).
- Implement pagination handling:
- If using numbered pagination links:
- Extract the total number of pages or the last page number.
- Iterate through the page range and construct the URL for each page.
- Send a request to each page URL and parse the response.
- If using a "Load More" button or infinite scrolling:
- Identify the API endpoint that returns the additional job listings.
- Send requests to the API endpoint with increasing offset or page parameters until no more results are returned.
- Parse the JSON or HTML response to extract the job data.
- If using numbered pagination links:
- Scrape and store data from each page:
- Use Beautiful Soup or a similar library to parse the HTML of each page.
- Extract the relevant job information, such as title, company, location, and description.
- Store the scraped data in a structured format (e.g., CSV, JSON) or database.
Here's a code snippet that demonstrates pagination handling using numbered pagination links:
import requests
from bs4 import BeautifulSoup
base_url = 'https://www.linkedin.com/jobs/search/?start={}'
start = 0
page_size = 25
while True:
url = base_url.format(start)
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract job data from the current page
job_listings = soup.find_all('div', class_='job-listing')
for job in job_listings:
# Extract and store job information
# ...
# Check if there are more pages
if not soup.find('a', class_='next-page'):
break
start += page_size
In this example, the script starts from the first page and iteratively sends requests to the subsequent pages by updating the start
parameter in the URL. It extracts the job listings from each page using Beautiful Soup and stops when there are no more pages to scrape.
By automating pagination handling, you can ensure that your LinkedIn scraper captures data from all available job listings, providing a comprehensive dataset for further analysis and utilization.
Bardeen can make web scraping easier. Use our extract LinkedIn job data playbook to boost your efficiency.
.png)
Saving and Utilizing Scraped LinkedIn Data
Once you have successfully scraped data from LinkedIn, the next step is to store and utilize the collected information effectively. There are several methods to save scraped data, depending on your specific requirements and the volume of data. Here are a few common approaches:
- CSV Files:
- Write the scraped data to a CSV (Comma-Separated Values) file using Python's built-in
csv
module. - Each row in the CSV file represents a single record, with columns corresponding to different data fields (e.g., job title, company, location).
- CSV files are simple, portable, and can be easily imported into spreadsheet applications or databases for further analysis.
- Write the scraped data to a CSV (Comma-Separated Values) file using Python's built-in
- Databases:
- Store the scraped data in a database management system like MySQL, PostgreSQL, or MongoDB.
- Use Python libraries such as
SQLAlchemy
orPyMongo
to interact with the database and insert the scraped records. - Databases provide structured storage, efficient querying, and the ability to handle large datasets.
- JSON Files:
- If the scraped data has a hierarchical or nested structure, consider saving it in JSON (JavaScript Object Notation) format.
- Use Python's
json
module to serialize the data into JSON strings and write them to a file. - JSON files are lightweight, human-readable, and commonly used for data interchange between systems.
When saving and utilizing scraped data from LinkedIn, it's crucial to consider the ethical and legal implications. LinkedIn's terms of service prohibit the use of scrapers or automated tools to extract data from their platform without explicit permission. Violating these terms can result in account suspension or legal consequences.
To ensure compliance with LinkedIn's policies, consider the following guidelines:
- Review and adhere to LinkedIn's terms of service and privacy policy.
- Obtain necessary permissions or licenses before scraping data from LinkedIn.
- Use the scraped data responsibly and in accordance with applicable data protection laws, such as GDPR or CCPA.
- Anonymize or aggregate sensitive personal information to protect user privacy.
- Avoid excessive or aggressive scraping that may strain LinkedIn's servers or disrupt the user experience.
By following ethical practices and respecting LinkedIn's terms of service, you can leverage the scraped data for various purposes, such as market research, talent acquisition, or competitor analysis, while mitigating legal risks.
Remember, the scraped data is only as valuable as the insights you derive from it. Analyze the collected information, identify patterns or trends, and use data visualization techniques to communicate your findings effectively. Combining the scraped LinkedIn data with other relevant datasets can provide a more comprehensive understanding of your target market or industry.
Automate LinkedIn Data Extraction with Bardeen
While scraping data from LinkedIn using Python requires a deep understanding of web scraping techniques and handling complex libraries, there's a simpler way. Automating data extraction from LinkedIn can be seamlessly achieved using Bardeen. This approach not only saves time but also enables even those with minimal coding skills to gather LinkedIn data effectively.
Here are examples of how Bardeen automates LinkedIn data extraction:
- Get data from a LinkedIn profile search: Perfect for market research or lead generation, this playbook automates the extraction of LinkedIn profile data based on your search criteria.
- Get data from the LinkedIn job page: Ideal for job market analysis or job search automation, this playbook extracts detailed job listing information from LinkedIn.
- Get data from the currently opened LinkedIn post: Enhance your content strategy or competitor analysis by extracting data from LinkedIn posts efficiently.
Streamline your LinkedIn data gathering process by downloading the Bardeen app at Bardeen.ai/download.
Explore top sales prospecting strategies and tips to identify potential customers and grow your business in 2024.
Learn to scrape LinkedIn data using Python, covering setup, libraries like Selenium, Beautiful Soup, and navigating LinkedIn's dynamic content.
Learn how to scrape LinkedIn data using R with web scraping techniques or the LinkedIn API, including steps, packages, and compliance considerations.
Learn how to scrape LinkedIn data using React, Python, and specialized tools. Discover the best practices for efficient data extraction while complying with legal requirements.
Learn to scrape LinkedIn using Beautiful Soup and Python for data analysis, lead generation, or job automation, while adhering to LinkedIn's terms of service.
Looking to download your own or another's LinkedIn profile picture? Discover how LinkedIn photo download can be easily done, with privacy top of mind.
Your proactive teammate — doing the busywork to save you time
Integrate your apps and websites
Use data and events in one app to automate another. Bardeen supports an increasing library of powerful integrations.
Perform tasks & actions
Bardeen completes tasks in apps and websites you use for work, so you don't have to - filling forms, sending messages, or even crafting detailed reports.
Combine it all to create workflows
Workflows are a series of actions triggered by you or a change in a connected app. They automate repetitive tasks you normally perform manually - saving you time.
Don't just connect your apps, automate them.
200,000+ users and counting use Bardeen to eliminate repetitive tasks